生成图像的缩略图
很多时候,在下载图像时,您不想保存完整图像,而只想保存缩略图。 或者您也可以保存全尺寸图像和缩略图。 可以使用 Pillow 库在 python 中轻松创建缩略图。 Pillow 是 Python 图像库的一个分支,包含许多用于操作图像的有用函数。 您可以在 https://python-pillow.org 找到有关 Pillow 的更多信息。 在本食谱中,我们使用 Pillow 创建图像缩略图。
准备工作
此菜谱的脚本是 04/07_create_image_thumbnail.py。 它使用 Pillow 库,因此请确保您已使用 pip 或其他包管理工具将 Pillow 安装到您的环境中:
pip install pillow
怎么做
以下是如何继续执行示例:
运行示例的脚本。 它将执行以下代码:
from os.path import expanduser
import const
from core.file_blob_writer import FileBlobWriter
from core.image_thumbnail_generator import ImageThumbnailGenerator
from util.urls import URLUtility
# download the image and get the bytes
img_data = URLUtility(const.ApodEclipseImage()).data
# we will store this in our home folder
fw = FileBlobWriter(expanduser("~"))
# Create a thumbnail generator and scale the image
tg = ImageThumbnailGenerator(img_data).scale(200, 200)
# write the image to a file
fw.write("eclipse_thumbnail.png", tg.bytes)
结果将是一个名为 eclipse_thumbnail.png 的文件写入您的主目录中。
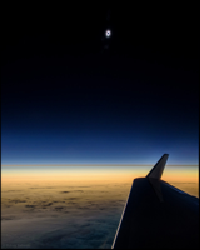
Pillow 保持宽度和高度的比例一致。
工作原理
ImageThumbnailGenerator 类包装对 Pillow 的调用,以提供一个非常简单的 API 来创建图像的缩略图:
import io
from PIL import Image
class ImageThumbnailGenerator():
def __init__(self, bytes):
# Create a pillow image with the data provided
self._image = Image.open(io.BytesIO(bytes))
def scale(self, width, height):
# call the thumbnail method to create the thumbnail
self._image.thumbnail((width, height))
return self
@property
def bytes(self):
# returns the bytes of the pillow image
# save the image to an in memory objects
bytesio = io.BytesIO()
self._image.save(bytesio, format="png")
# set the position on the stream to 0 and return the underlying data
bytesio.seek(0)
return bytesio.getvalue()
构造函数会传递图像的数据,并根据该数据创建一个 Pillow 图像对象。 缩略图是通过使用表示所需缩略图大小的元组调用 .thumbnail() 创建的。 这会调整现有图像的大小,并且 Pillow 会保留纵横比。 它将确定图像的较长边并将其缩放为表示该轴的元组中的值。 该图像的高度大于宽度,因此缩略图的高度为 200 像素,宽度也相应缩放(在本例中为 160 像素)。