模块化编程
Node.js 主要使用模块系统进行编程,所谓模块,是指为了方便调用功能,预先将相关方法和属性封装在一起的集合体。模块和文件是一一对应的,即一个 Node.js 文件就是一个模块,这个文件可以是 JavaScript 代码、JSON
或者编译过的 C/C++ 扩展等。下面对 Node.js 模板化编程中用到的两个对象 exports
和 module
进行讲解。
exports 对象
在 Node.js 中创建模块需要使用 exports
对象,该对象可以共享方法、变量、构造和类等,下面通过一个实例讲解如何使用 exports
创建一个模块。
【例4.2】使用 exports
对象实现模块化编程。(实例位置:资源包\源码\04\02)
步骤如下。
(1) 在 WebStorm
中创建一个 module.js
文件,其中通过 exports
对象共享求绝对值和计算圆面积的方法,代码如下:
//求绝对值的方法abs
exports.abs = function (number) {
if (0 < number) {
return number;
} else {
return -number;
}
};
//求圆面积的方法circleArea
exports.circleArea = function (radius) {
return radius * radius * Math.PI;
};
(2) 在 WebStorm
中创建一个 main.js
文件,用来调用前面创建的模块来计算指定值的绝对值及指定半径的圆面积,代码如下:
//加载module.js模块文件
var module = require('./module.js');
//使用模块方法
console.log('abs(-273) = %d', module.abs(-273));
console.log('circleArea(3) = %d', module.circleArea(3));
上面代码中,通过使用 |
运行 main.js
文件,结果如下:
abs(-273) = 273
circleArea(3) = 28.274333882308138
module 对象
在 Node.js 中,除了使用 exports
对象进行模块化编程,还可以使用 module
对象进行模块化编程。module
对象的常用属性如表4.7所示。
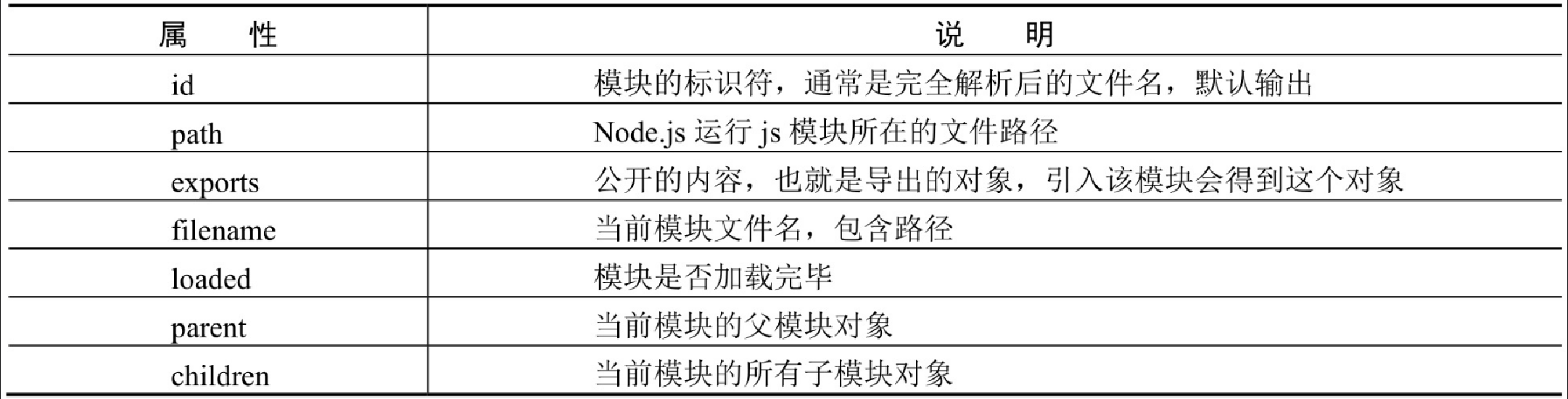
【例4.3】使用 module
对象实现模块化编程。(实例位置:资源包\源码\04\03)
步骤如下。
(1) 在 WebStorm
中创建一个 module.js
文件,其中定义一个输出方法,然后通过 module
对象的 exports
属性指定对外接口,代码如下:
function Hello() {
var name;
this.setName = function (thyName) {
name = thyName;
};
this.sayHello = function () {
console.log(name + ',你好');
};
};
module.exports = Hello;
(2) 在 WebStorm
中创建一个 main.js
文件,用来调用创建的模块以输出内容,代码如下:
var Hello = require('./module.js');
hello = new Hello();
hello.setName('2023');
hello.sayHello();
运行 main.js
文件,结果如下:
2023,你好
与使用 |