数据属性 (Props)
数据(data)属性是构建 Vue 组件时最常用的术语和响应式元素之一。这些属性体现在 Vue 实例的数据功能中:
<template>
<div>{{color}}</div>
</template>
<script>
export default {
data() {
return {
color: 'red'
}
}
}
</script>
您可以使用 data 属性来存储您想要在 Vue 模板中使用的任何信息。当此数据属性更新或更改时,它将在相应的模板中进行响应式更新。
练习 1.01:构建你的第一个组件
在本练习中,我们将在 Vue 项目中构建第一个组件。在这种情况下,组件是使用 ES6
语法导入的。我们需要安装 Node.js 和 YARN
。这些将在前言中介绍。练习结束时,您将能够自信地使用 Vetur(是一个 VS Code 上的 Vue 插件) 创建新的 Vue 组件并将其导入到您的项目中。
要访问本练习的代码文件,请参阅 https://packt.live/35Lhycl 。
-
打开命令行终端并导航到 Exercise 1.01 文件夹并按顺序运行以下命令:
> cd Exercise1.01/ > code . > yarn > yarn serve
当您保存新更改时,您的应用程序将热重载,以便您可以立即看到它们。
-
在
VSCode
(当您运行code .
命令时它会打开)中,进入src/App.vue
目录并删除该文件中的所有内容并保存。 -
在您的浏览器中,一切都应该是空白的,需要从头开始工作。
-
构成单文件组件的三个主要组件是
<template>
、<script>
和<style>
块。如果您在前面已经安装了Vetur
扩展,请输入vue
并按 Tab 键选择提示符的第一个选择。这是设置默认代码块的最快方法,如以下屏幕截图所示:Figure 1. Figure 1.5: VSCode Vetur以下是使用 Vetur 时按 Tab 后生成的代码:
// src/App.vue <template> </template> <script> export default { } </script> <style> </style>
-
在
components
文件夹中创建另一个名为Exercise1-01.vue
的文件,并重复相同的步骤,使用 Vetur 搭建 Vue 块:// src/components/Exercise1-01.vue <template> </template> <script> export default { } </script> <style> </style>
-
在我们的
Exercise1-01.vue
组件中,组成一组<div>
标签,在<template>
标签内包含一个<h1>
元素和一个标题:<template> <div> <h1>My first component!</h1> </div> </template>
-
在
<style>
块内,添加一些样式,如下所示:<template> <div> <h1>My first component!</h1> </div> </template> <style> h1 { font-family: 'Avenir', Helvetica, Arial, sans-serif; text-align: center; color: #2c3e50; margin-top: 60px; } </style>
-
使用 ES6
import
方法将组件导入App.vue
,并在<script>
块的components
对象中定义组件。现在,我们可以在 HTML 中以camelCase
或kebab-case
引用该组件的名称(两者均可):
<template>
<Exercise />
</template>
<script>
import Exercise from './components/Exercise1-01'
export default {
components: {
Exercise,
}
}
</script>
当您按 “保存” 时,https://localhost:8080 应重新加载并显示以下输出:
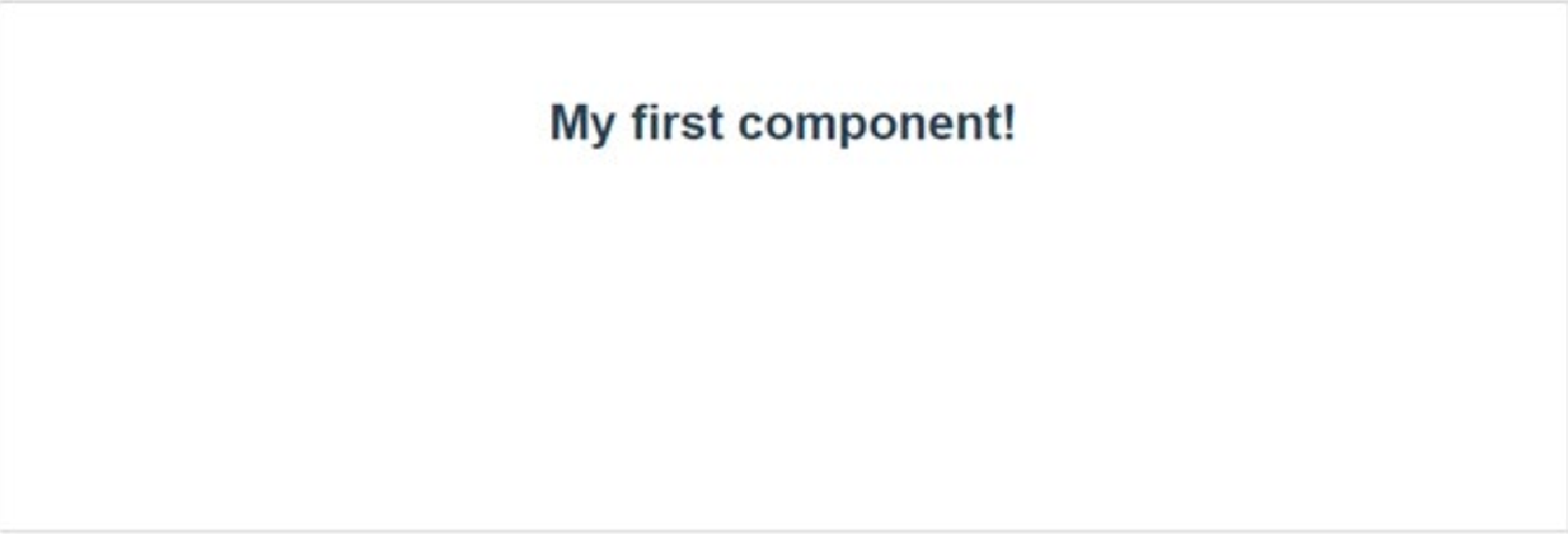
在本练习中,我们了解了如何使用模板标签构建 Vue 组件,使用 Vetur
构建基本 Vue 组件,输出 HTML,以及使用 ES6 语法将 Exerce1-01
组件导入 App.vue
。
|