深度观察(Watch)概念
当使用 Vue.js 观察数据属性时,您可以有目的地观察对象内部的键是否发生变化,而不是对象本身的变化。这是通过将可选的 deep
属性设置为 true
来完成的:
data() {
return {
organization: {
name: 'ABC',
employees: [
'Jack', 'Jill'
]
}
}
},
watch: {
organization: {
handler: function(v) {
this.sendIntercomData()
},
deep: true,
immediate: true,
},
},
此示例将监视 organization 数据对象内的所有可用键是否发生更改,因此如果组织内的 name 属性发生更改,organization 观察器将触发。
如果不需要观察对象内的每个键,则通过将其指定为 myObj.value
字符串来观察对象内的特定键的更改可能会更高效。例如,您可以允许用户编辑其公司名称,并仅在修改该键后才将该数据发送到 API。
在下面的示例中,观察者专门观察 organization 对象的 name 键。
data() {
return {
organization: {
name: 'ABC',
employees: [
'Jack', 'Jill'
]
}
}
},
watch: {
'organization.name': {
handler: function(v) {
this.sendIntercomData()
},
immediate: true,
},
},
我们看到了深度观察的作用。现在,让我们尝试下一个练习并观察数据对象的嵌套属性。
练习2.04:观察数据对象的嵌套属性
在本练习中,您将使用观察程序来观察对象中的键,当用户触发 UI 中的方法时,该对象将更新。
要访问本练习的代码文件,请参阅 https://packt.live/353m59N 。
-
打开命令行终端,导航到 Exercise 2.04 文件夹,然后按顺序运行以下命令:
> cd Exercise2.04/ > code . > yarn > yarn serve
-
首先定义一个包含 price 和 label 以及 discount 键的 product 对象。将这些值输出到模板中:
<template> <div class="container"> <h1>Deep Watcher</h1> <div> <h4>{{ product.label }}</h4> <h5>${{ product.price }} (${{ discount }} Off)</h5> </div> <br /> <a href="#" @click="updatePrice">Reduce Price!</a> </div> </template> <script> export default { data() { return { discount: 0, product: { price: 25, label: 'Blue juice', }, } }, } </script> <style lang="scss" scoped> .container { margin: 0 auto; padding: 30px; max-width: 600px; font-family: 'Avenir', Helvetica, sans-serif; margin: 0; } a { display: inline-block; background: rgb(235, 50, 50); border-radius: 10px; font-size: 14px; color: white; padding: 10px 20px; text-decoration: none; } </style>
-
设置一个可以修改产品价格的按钮。通过添加一个带有绑定到
updatePrice
方法的click
事件的按钮元素来实现此目的,该方法会减少价格的值:<template> ... <a href="#" @click="updatePrice">Reduce Price!</a> ... </template> <script> ... methods: { updatePrice() { if (this.product.price < 1) return this.product.price-- }, }, ... </script>
当您单击该按钮时,它应该会降低价格,如以下屏幕截图所示:
Figure 1. Figure 2.6: Screen displaying the reduced price of Blue juice -
嵌套观察者的时间到了。我们将观察 product 对象的 price,并增加 discount 数据属性:
watch: {
'product.price'() {
this.discount++
},
},
现在,当你降低 price 时,由于观察者的原因,discount 值将会上升:
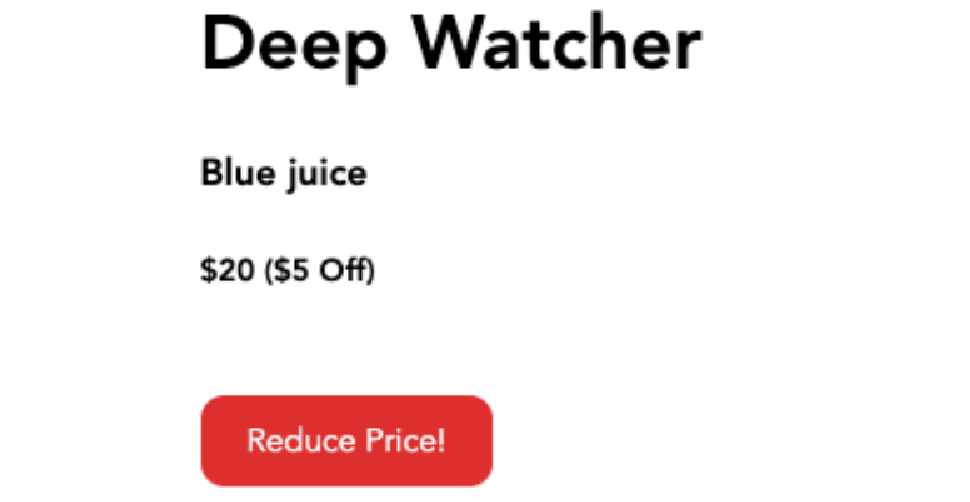
在本练习中,我们使用观察程序来观察对象内的键,然后使用或不使用观察程序解析的可选参数来设置新数据。