计算属性
计算属性是一种独特的数据类型,当属性中使用的源数据更新时,它将进行响应式更新。它们可能看起来像 Vue 方法(method),但事实并非如此。在 Vue 中,我们可以通过将数据属性定义为计算属性来跟踪数据属性的更改,在此属性中添加自定义逻辑,并在组件中的任何位置使用它来返回值。计算属性会被 Vue 缓存,这使得它们在返回数据方面比 data 属性或使用 Vue 方法(method)具有更高的性能。
您可以使用计算属性的实例包括但不限于:
- 表单验证
-
在此示例中,当
total
数据属性小于 1 时,将出现一条错误消息。每次将新数据添加到items
数组时,total
的计算属性都会更新:
<template>
<div>{{errorMessage}}</div>
</template>
<script>
export default {
data() {
return {
items: []
}
},
computed: {
total() {
return this.items.length
},
errorMessage() {
if (this.total < 1) { // 这里调用其它计算属性
return 'The total must be more than zero'
} else {
return ''
}
}
}
}
</script>
这将生成以下输出:The total must be more than zero
- 组合数据属性
-
在以下示例中,您可以使用计算属性将两部分数据组合成一个可返回字符串,
formalName
,该字符串可在组件中使用:<template> <div>{{ formalName }}</div> </template> <script> export default { data() { return { title: 'Mr.', surname: 'Smith' } }, computed: { formalName() { return this.title + ' ' + this.surname } } } </script>
这将生成以下输出:Mr. Smith
- 将复杂信息输出到 Vue 模板中
-
在这个更复杂的示例中,我们使用计算属性来分解称为 post 的大数据对象。您将使用简化的语义计算属性将信息输出到组件模板中。此示例中的计算属性使您可以更轻松地识别和使用作者的全名,查看他们生成了多少帖子,并使用数据来显示他们的精选帖子:
<template>
<div>
<p>{{ fullName }}</p>
<p>{{ totalPosts }}</p>
<p>{{ featuredPosts }}</p>
</div>
</template>
<script>
export default {
data() {
return {
post: {
fields: {
author: {
firstName: 'John',
lastName: 'Doe'
},
entries: [{
title: "Entry 1",
content: "Entry 1's content",
featured: true
},
{
title: "Entry 2",
content: "Entry 2's content",
featured: false
}]
}
}
}
},
computed: {
fullName() {
// Return string
return this.post.fields.author.firstName + ' ' +
this.post.fields.author.lastName
},
totalPosts() {
// Return number
return this.post.fields.entries.length
},
featuredPosts() {
// Return string
return this.post.fields.entries.filter(entry => {
// If featured is true, return the entry title
if (entry.featured) {
return entry
}
})
}
}
}
</script>
这将生成以下输出:
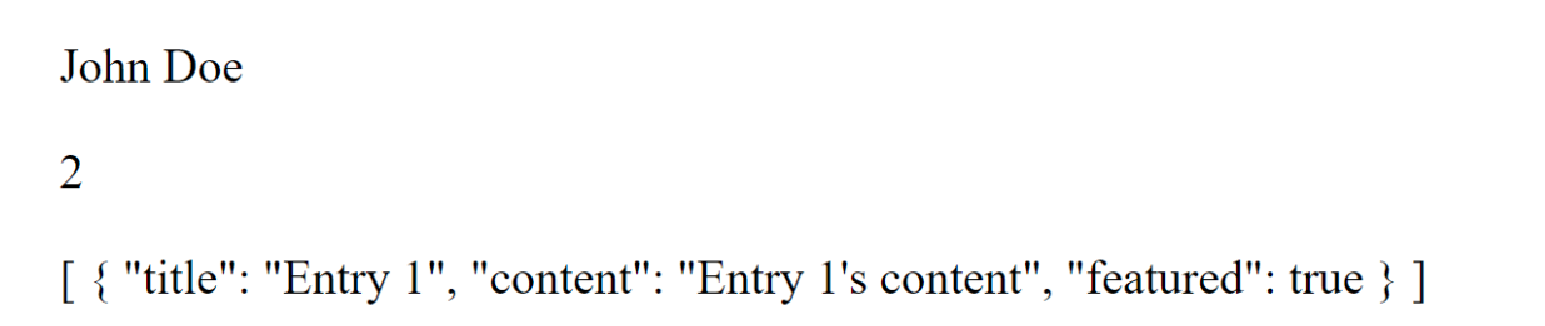
在创建高性能组件时,计算属性对于 Vue 开发人员非常有价值。在下一个练习中,我们将探索如何在 Vue 组件内部使用它。
练习 2.01:将计算数据实现到 Vue 组件中
在本练习中,您将使用计算属性通过简洁地输出基本数据来帮助减少需要在 Vue 模板中编写的代码量。要访问本练习的代码文件,请参阅 https://packt.live/3n1fQZY 。
-
打开命令行终端,导航到 Exercise 2.01 文件夹,然后按顺序运行以下命令:
> cd Exercise2.01/ > code . > yarn > yarn serve
-
为名字创建一个输入字段,使用
v-model
将数据属性firstName
绑定到该字段:<input v-model="firstName" placeholder="First name" />
-
为姓氏创建第二个输入字段,并使用
v-model
将数据属性lastName
绑定到该字段:<input v-model="lastName" placeholder="Last name" />
-
通过在
data()
函数中返回这些新的v-model
数据属性,将它们包含在 Vue 实例中:data() { return { firstName: '', lastName: '', } },
-
创建一个名为
fullName
的计算数据变量:computed: { fullName() { return `${this.firstName} ${this.lastName}` }, },
-
在输入字段下方,使用 heading 标签输出计算出的数据:
<h3 class="output">{{ fullName }}</h3>
这将生成以下输出:
Figure 2. Figure 2.2: Output of the computed data will show the first and last name
在本练习中,我们了解了如何使用 v-model
中的数据在计算数据属性内编写表达式,并将名字和姓氏组合到可重用的单个输出变量中。